What is the Fibonacci Series?
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, starting from 0 and 1. The sequence goes 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
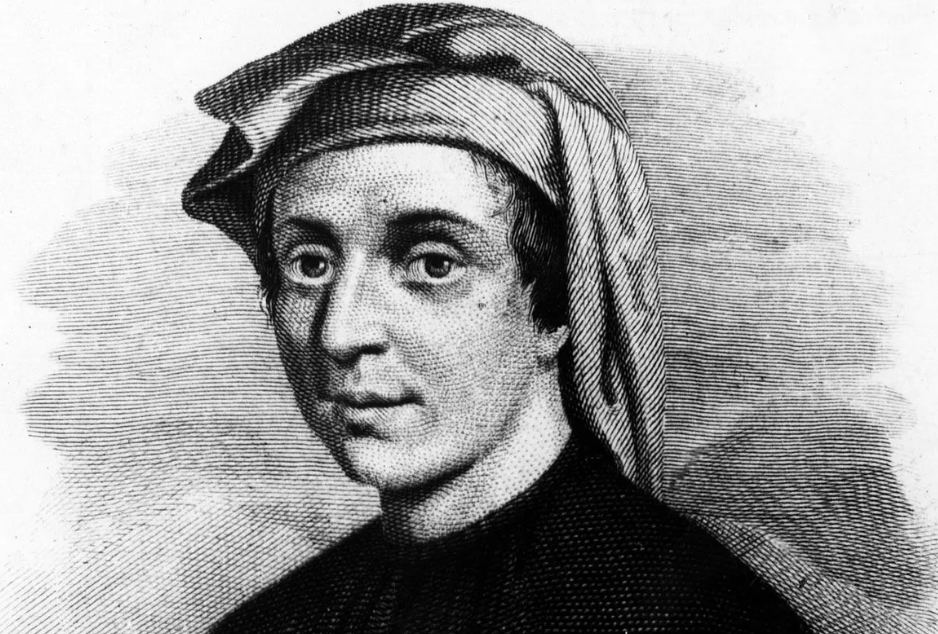
The Fibonacci sequence is named after the Italian mathematician Leonardo of Pisa, who was known as Fibonacci. It is not just a mathematical sequence, but has several applications in various fields such as nature, art, music, and financial markets.
In mathematics, it is used to illustrate the growth of systems that follow a recursive pattern. In biology, it appears in the arrangement of leaves, branches, and flowers on plants. In finance, it is used to identify potential re-tracement levels in stock prices.
Overall, the Fibonacci sequence provides a simple example of a pattern that occurs in many different areas of science and nature, making it a popular topic in mathematics education and research.
Now lets walk through the code
To note: the Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. Once you understand you could just build this algorithm without a walkthrough. But if you want to save your time here is the answer!
- To write a Java program to print a Fibonacci series:
- Initialize the number of elements to print (
n
) - Initialize two variables (
a
andb
) to the first two numbers in the series (0 and 1) - Use a loop to print the first
n
numbers in the series:- Print the current value of
a
- Compute the next number in the series by adding
a
andb
- Update the values of
a
andb
so thata
becomes the previous value ofb
, andb
becomes the current value of the sum
- Print the current value of
- Output the series
- Initialize the number of elements to print (
- The program could be modified to take input from the user for the number of elements to print, or to use recursion to generate the series
Code example
public class FibonacciSeriesExample {
public static void main(String[] args) {
int n = 10; // number of elements to print
int a = 0, b = 1;
System.out.print("Fibonacci Series up to " + n + " terms: ");
for (int i = 1; i <= n; i++) {
System.out.print(a + " ");
int sum = a + b;
a = b;
b = sum;
}
}
}
Final Note
The Fibonacci sequence is a fundamental concept in mathematics and computer science, and is used in many different applications. Understanding how to code the Fibonacci sequence is important for developing problem-solving skills and algorithmic thinking. Coding the Fibonacci sequence is a useful exercise for beginners in programming, as it helps to build understanding of basic programming concepts such as loops, variables, and data types. Knowledge of how to code the Fibonacci sequence can be applied in many other areas of programming, such as developing algorithms for financial modelling, image processing, and cryptography. In addition, the Fibonacci sequence is found in many natural phenomena, such as the spiral patterns of seashells and sunflowers, and understanding its properties can help us better understand the world around us. Finally, being able to code the Fibonacci sequence is a valuable skill for anyone interested in pursuing a career in computer science, mathematics, or related fields, as it is a commonly used example in interviews and programming challenges.